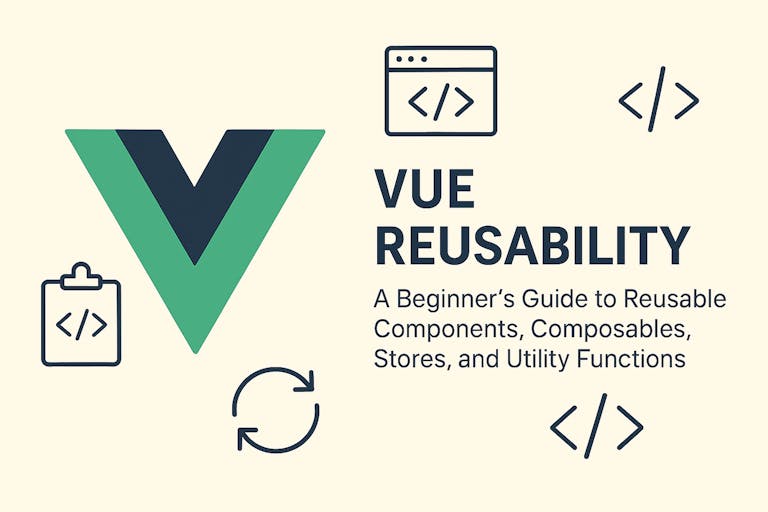
A Beginner's Guide to Reusability in Vue
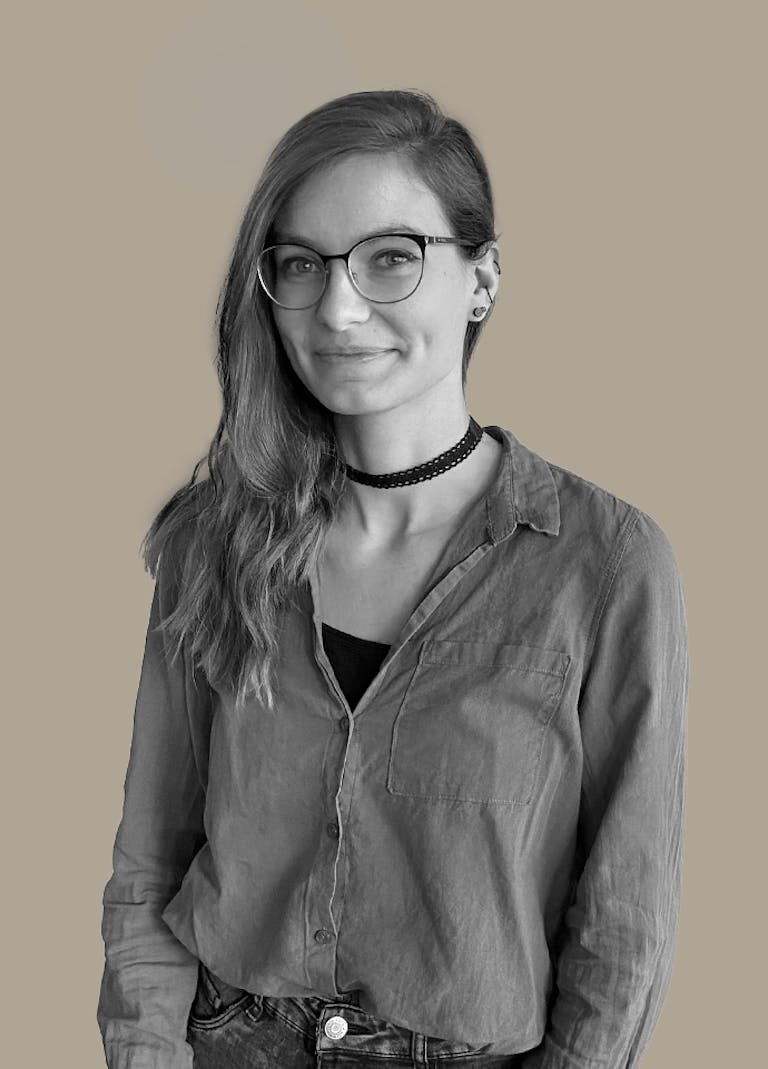
Lea
April 4, 2025
Intro
One of the most important aspects in having clean, maintainable software is reusability, or as many developers say, following the DRY (Don't Repeat Yourself) principle. Reusing code means we have less to maintain, making the system less error-prone. This is true for all kinds of coding, no matter the technology or the framework. And it's probably also true for a lot of other professions. Today we want to specifically talk about one framework: Vue.js. This article won’t focus on why or when to use reusable code. Instead, we'll look at which approach to use in Vue.
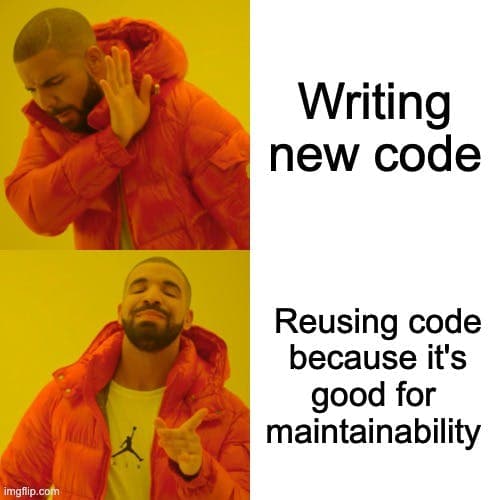
I'm calling this a beginners guide, because a lot of these principles aren't new or groundbreaking. In reality, you should know these if you developed a bit already. But because reality is not perfect, I've read quite a bit of code that doesn't follow these guidelines. So this is mainly for following people:
- beginners who are new to developing with frameworks
- people that come from other frameworks and want to get into Vue's opinionated development
- when you're just not sure about what reusable concept you should use
- to send a link to someone who doesn’t follow these practices :)
Vue is an opinionated framework and gives us several options for reusing code. The examples in this article use the Vue Composition API. Not all of the approaches are suitable for every scenario. Let's start with the most obvious one: Components.
Components - The Visible One
We've all used components. And if you haven't used one yet, what are you doing?? The key for a consistent behaviour throughout your app is using components that encapsulate small parts of your UI. Components are often written to follow the principles of Atomic Design. We have atoms like buttons, inputfields or icons, then molecules like search fields, organisms built from these and so on. Vue uses Single File Components (SFCs), which encapsulate template, script and style into one file. Vue requires a SFC to have at least a template or a script tag. So when should we use components for reusable logic?
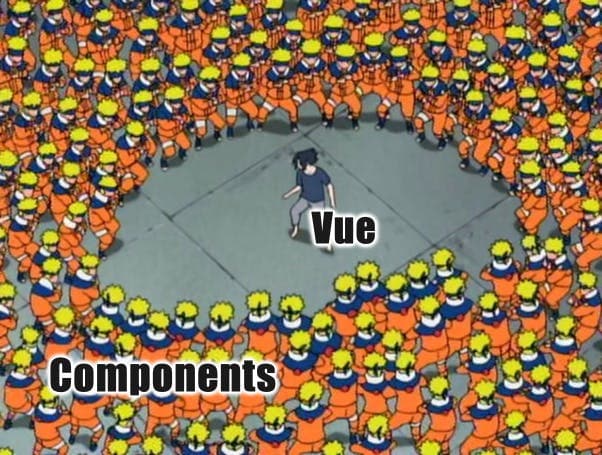
Components should always implement a specific part of the UI, that means:
- a component should always have a template tag
- the logic in the component shouldn't need to be reused anywhere else
- make sure the styling is also scoped
The naming convention for Vue components are Multiword titles.
Examples: TextButton, ReadonlyTable, TextInput, ModalDialog, SectionWrapper, CustomForm
Scoped styling is a blessing, and should be used whenever possible. A scoped styling makes sure that the styling is only applied to the component, in which it was defined and doesn't leak to other components. It makes reusing components so much easier, because you know what styling to expect and don't have to check any other files for the big question: why is this not centered? Sometimes, adjusting the styling of child components is unavoidable. In such cases, use :deep(.class) so the change is not global to the whole application but only for this component and the ones below it in the dependency tree.
Another concept for components that makes reusing these so simple is slots. A parent can give HTML content to a child component and it's rendered in place of the slot tag. Not all components need this, but this way you can even build container components, like a wrapper for a card or a modal. You can import components either locally or globally. If you’re unsure which one to choose, always prefer local imports first. That way the code is only included in the bundle if you use them. Global usage only makes sense if you are sure you will need them in a lot of components, most often this is only used to include libraries. You can also include common basic components, if you think explicitly importing them increases your codelines too much. (I never do that to be honest, but I also don't want to step on anyones toes that does. You do you.)
The following example shows a basic implementation of a button in action. Nothing fancy, really.
Composables - The Fancy One
Some people might argue that you can write a component without a template tag and instead only include a script tag. This will still compile and is not wrong per se. But we can use something better for this: Composables. A composable is a file that exports a function containing logic. If you already implemented with Vue 2 you may know the old variant of this concept, which was called mixins. As mixins had a lot of problems, Vue 3 gave us this new version. A composable only includes logic, there is no template or styling included. If you don't need these, you should always use a composable over a component with only a script tag. The reason is that composables don’t require the overhead of a renderless component instance. Each component has a lifecycle and tracks reactivity, whereas a composable is simply a JavaScript function that tracks reactivity only for the reactive variables it uses.
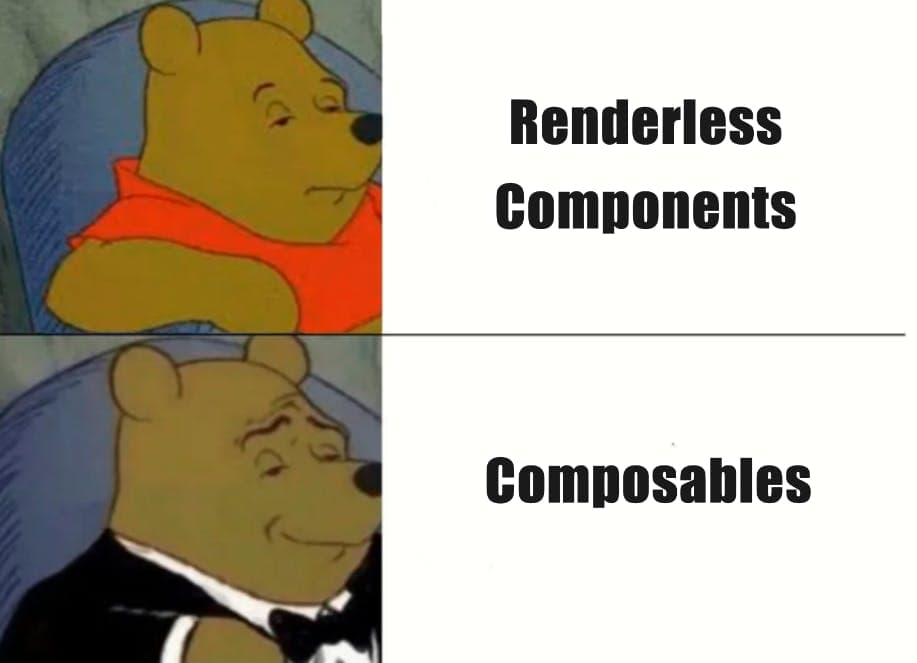
You should use a composable if:
- you need reusable logic
- there is no reason for template or styling
- you need to manage state for this logic (we're going to talk about stateless logic later)
- you want to use Vue API methods*
- the state shouldn't be shared between components that are using the same composable
Let's talk a bit more about the last point. Some small applications might want to manage their fetching logic without a library and write a composable useFetch for it. The file has a parameter queryand exports the result data and the loading state. You can use the composable in each file you want to fetch something from a backend. But each usage should return the result of the query you asked for and the loading state for this query. If ComponentA with the composable has loading: true, ComponentB may already have completed the request and show loading: false. Even if both components use the same parameter simultaneously, their states remain separate. That means each usage should be scoped to the component. The downside of composables is, that they are written for the Composition API, which means we need a setup function to use them. You can add a setup hook to the Options API, too, but you should be aware, that the initialization of data happens after the setup. The naming convention for composables is useSomething.
Examples: useFetch, useMouse, useCalculation
Vue also curates a large collection of useful composition utilies, that are written as composables at VueUse. Consider using these before writing basic composables such as using localStorage, the Browser API or mathematical calculations.
Here you can see an example in action. The goal is to have a reusable composable, that gets an HTML Element and returns its size.
Store - The Shared One
We just talked about stateful logic limited to the instance of a component. Sometimes, we need to share state across components, pages, and composables. Using a composable for these cases could lead to unnecessary performance overhead and memory leaks, e.g. if you write a composable to track the time you need to use setIntervalto get updates for the current time. Then you instantiate it for every single component where it's used. Instead you want to do this just one time, and reuse this across your application. To do this, you should use a store. Vue recommends Pinia for State management. Older users may know VueX, which is the predecessor to it. You can include logic in a store, but to honor the separation of concerns principle, it should correlate to the state. Keep logic related to state inside the store but extract complex, reusable logic into composables. If you want to use Vue API functions like watchEffecte.g. you can use Pinias setup store syntax.
You should use a store for
- shared state
The naming convention for stores is contentStore. The naming convention for stores is:
- useContentStore for the exported function that defines the store
- content.js for the file that contains the store
Examples: userStore, sessionStore
Utils - The Classic One
To finish our basic list, we also need some way to reuse stateless logic. Vue doesn't implement any special usage for this. Same as in any other project and when working without a framework, stateless logic should be held in utility files. Utility functions are independent of Vues reactivity system. You can also use libraries for these instead of writing them yourself. Lots of projects use e.g. lodash for utilities or date-fns to work with dates.
Utils are perfect for:
- stateless logic
- when you don't need any Vue API functions
- basically functions that receive an input and return an output
Examples: sortByCustom, calculateX, formatToY
Summary
Choosing the right reusability pattern in Vue is key to writing maintainable, scalable, and efficient code. Components handle UI elements, composables manage reusable logic, stores maintain shared state, and utility functions simplify stateless operations. Below you can see a table that summarizes the difference in usage in a visual way. Not all of these are set in stone, but you should be aware of them. If you want to go against these guidelines, there should be a valid reason for it.
Components | Composables | Store | Utils | |
---|---|---|---|---|
HTML/template tag | ✅ | ❌ | ❌ | ❌ |
Include styling | 🆗 | ❌ | ❌ | ❌ |
Implement logic | 🆗 | ✅ | 🆗 | ✅ |
Uses Vue API functions* | 🆗 | 🆗 | 🆗 | ❌ |
Shared State | ❌ | ❌ | ✅ | ❌ |
Reactivity | ✅ | 🆗 | ✅ | ❌ |
Ideal use case | UI elements | Reusable logic | Shared state | Pure functions |
✅ = yes ❌ = no 🆗 = optional
*exports like ref and reactive, compiler macros like defineProps, or helpers like watch as well as hooks etc.
As you can see, there are specific usecases for the different concepts. Using the correct one increases performance and ensures you have a clean and maintainable code, that other people can understand as well. Most of these concepts are similar to concepts in other frameworks. Components are a common concept. Composables for example are the same idea as hooks in React. And even though this article is about reusability, you can use all of these even if you don't want to reuse the code and just separate your files into smaller, more readable ones. Knowing when to split your code requires some practice, but understanding these points is the first step to be able to use the theory in reality.
Vue
JavaScript
Reusability
DRY Principle
Components
Read also
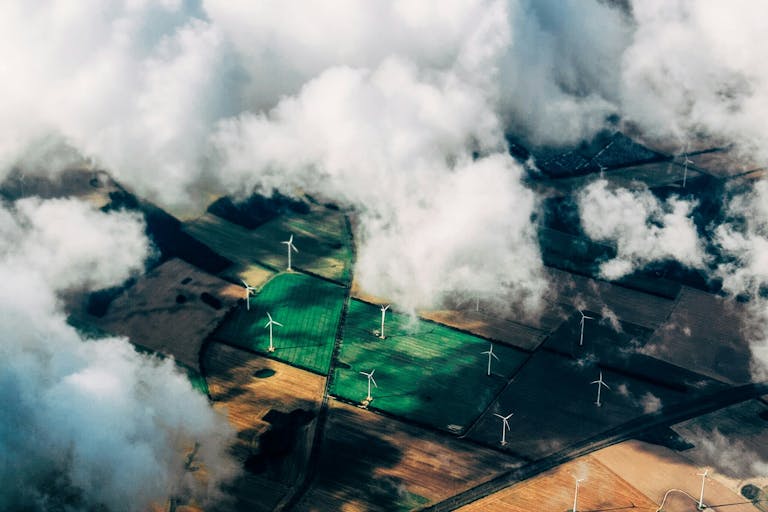
Michael, 04/16/2025
Green Hosting: A Sustainability Comparison
Sustainability
Green Software
Consulting
Hostingsec

Francesca, Ricarda, 04/02/2025
Top 10 Mistakes to Avoid When Building a Digital Product
MVP development
UX/UI design
product vision
agile process
user engagement
product development
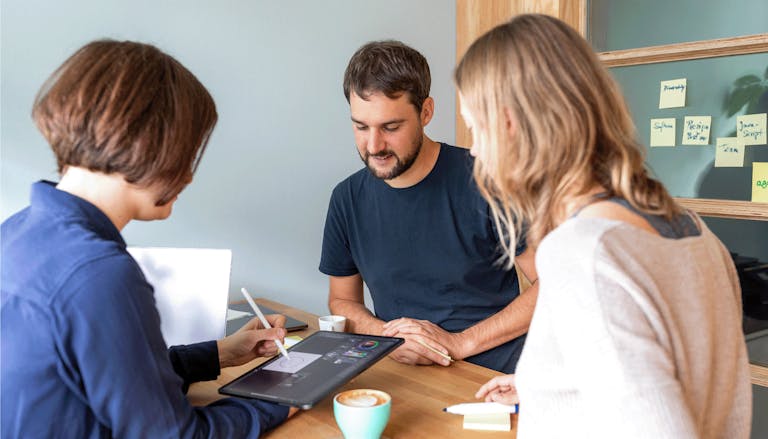
Judith, 03/31/2025